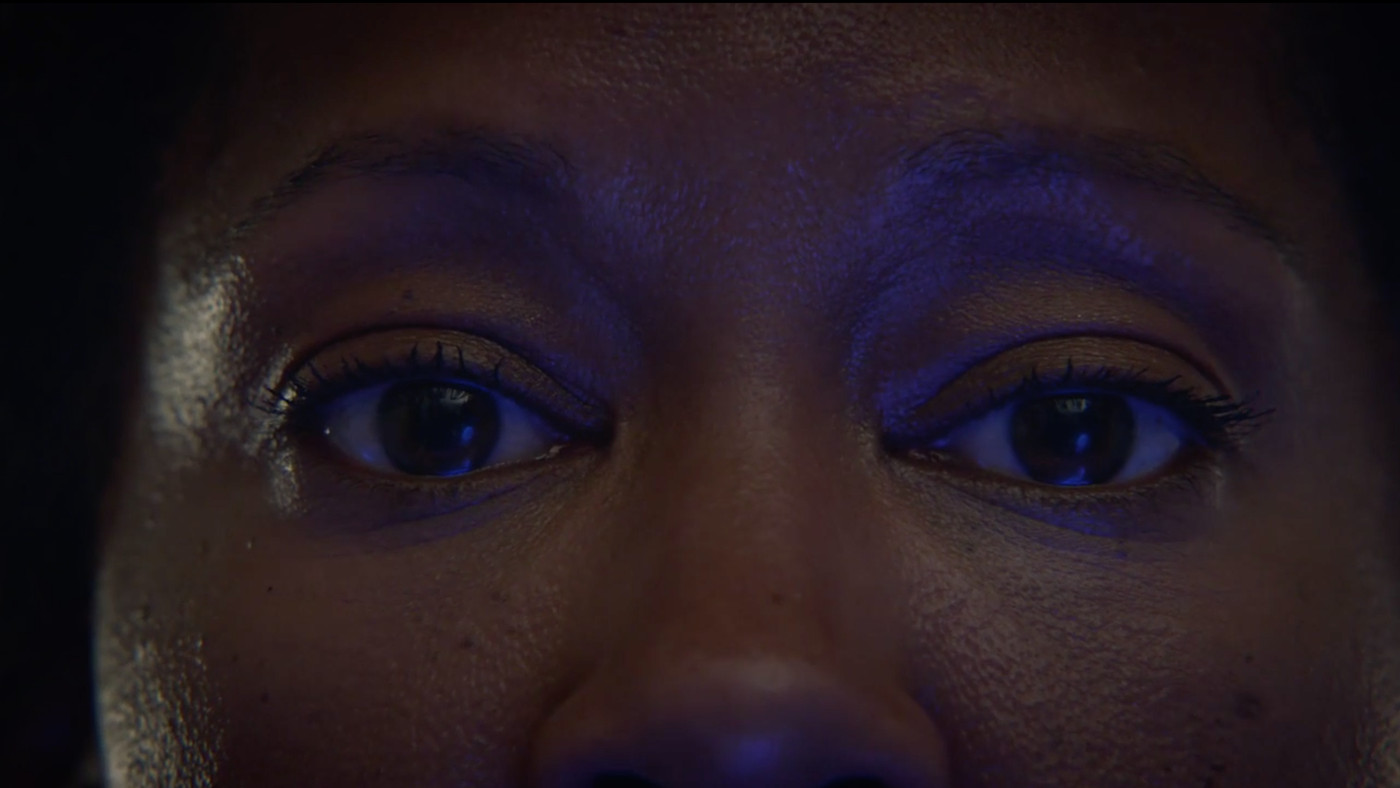
^^^ me opening up my laundry room door now
you probably opened up this post and expected to read about foods at various restaurants relative to their lighting. apologies, this is not that. in fact, this is much more about light than food...and its barely about light. Let me explain.
why would you not add a light.
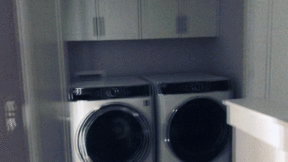
out of all the places someone could cut corners when building a home, i would've expected not adding a light fixture to the laundry room to be near the bottom of the list. i was wrong. sure, the lights in the hallways help illuminate the room a touch but still a far cry from what i wanted. plus i wanted an excuse to work on another project. enter openlight.
openlight
i wanted a simple solution to my no-light-laundry-room situation that 1) wouldn't cost me an arm and a leg to have installed (e.g. having an electrician wire this room up), 2) would require virtually no maintenance once installed, and 3) wouldn't require me to flip any switch when opening the laundry room door. i wanted the ability to just open the doors and voila - the lights are on. cool...so sounds like i should just buy three or four motion sensor lights from walmart for $13.98 each and call it a day, right? but why do that when you could build something yourself for 4x the cost and 2x the bugs!
brainstorming
what would be the most elegant solution to this problem? building motion detecting lights would definitely satisfy all three of my requirements...but i'm looking for a nbd ('never been done') solution. also i had one of these leftover simplisafe entry sensors that i wanted hack/modify:
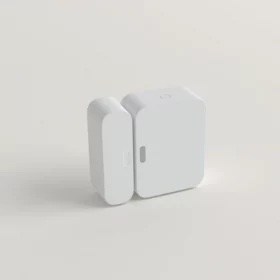
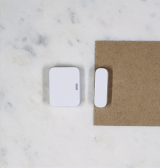
the entry sensor is composed of two magnets which, when separated by a certain distance, triggers a data transmission event to the simplisafe base station to alert of a door opening/closing. the entry sensor will also flash a blue indicator light to signal the trigger has been tripped.
#1
magnets separate from each other
#2
entry sensor trigger tripped and data sent to base station
#3
base station receives and acknowledges
here was my plan of attack:
- figure out how entry sensor transmits data to simplisafe home device
- intercept that data transmission to set the state of laundry door (either open or closed)
- turn a light on or off dependent upon laundry door state
- when complete, play JumpOutTheHouse by carti
step 1: simplisafe data transmission
so how was i going to figure out how and what the entry sensor was transmitting data to the base station? excellent question, and to answer it fully we need to have a quick history lesson.
1864 - james clerk maxwell publishes paper theorizing existence of electromagnetic waves propogating through free space, ultimately laying the groundwork for intentional transmission of information through use of these electromagnetic waves
1888 - heinrich hertz publishes results of his experiment showing transmission of electromagnetic waves through the air, validating maxwell's prediction
1900 - after multiple years of experimentation from various scientists/mathematicians, the first transmission of voice over airways occurred via radio communication by canadian-born inventor reginald fessenden. what was heard on the other end? "lose my number"
1933 - legal limits imposed on all digital equipment emitting electromagnetic waves in the u.s. brought by the federal communications commission ('fcc')
1975 - the fcc established regulations on electromagnetic emissions and the fcc mark is born. generally speaking, if anyone wants to release a commercial product that transmits electromagnetic waves, they need to get cleared by the fcc.
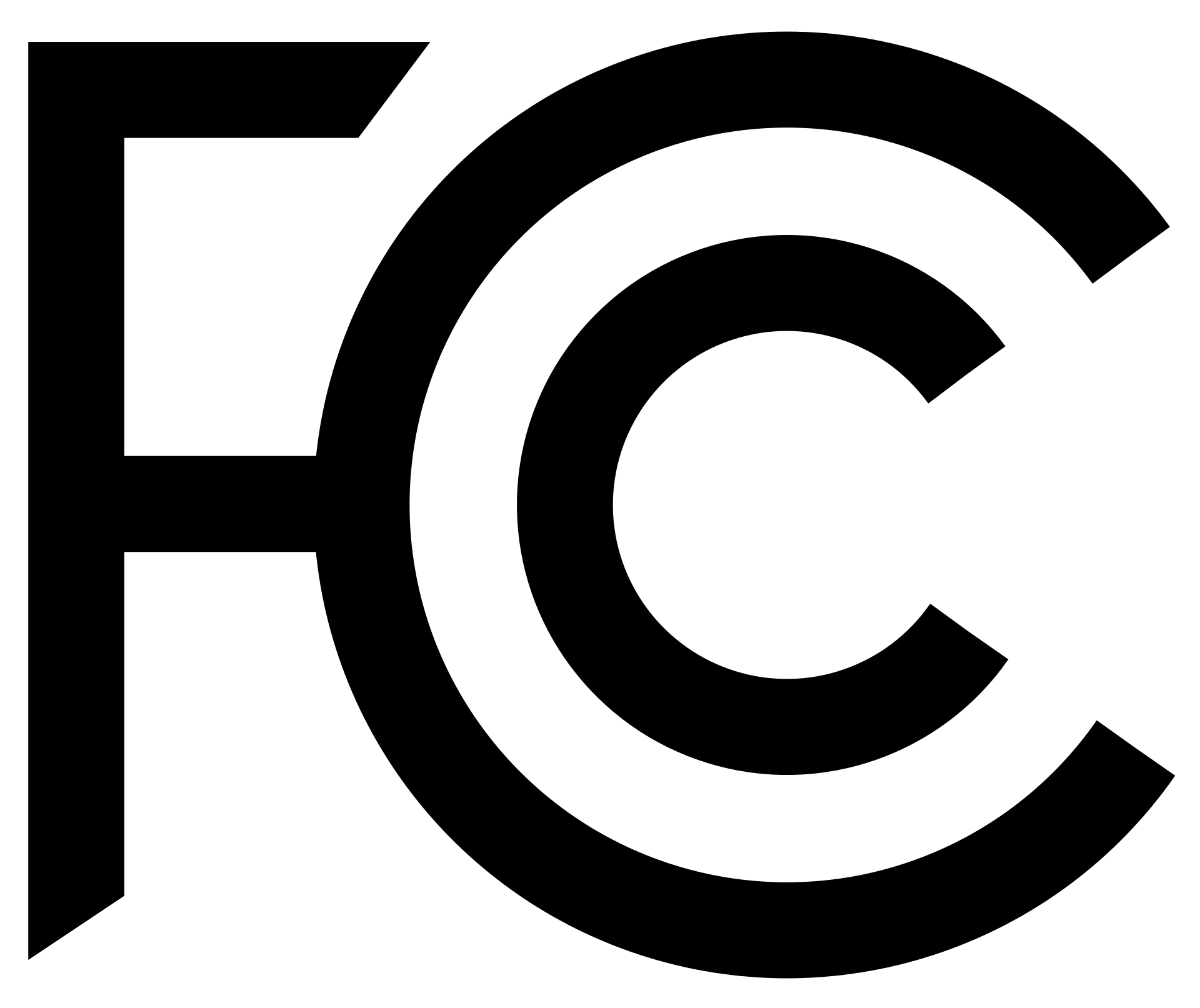
2017 - the fcc mark is no longer mandatory. but companies still submit paperwork to receive fcc validation.
tldr/what does this mean. it means that companies need to submit information about any device to the fcc in order to receive a fcc mark, or validation that their product's electromagnetic interference is under a certain threshold. the good news is that all of this information is publicly available. that means i can find out how the entry sensor is transmitting its data over the air. boom.
soooo based on that information the entry sensor is broadcasting its information at 433.92MHz. sweet, only EVER OTHER CONSUMER ELECTRONICS DEVICE broadcasts information at that frequency band. let's cross our fingers and hope for minimal interference?
step 2: let’s buy the tools
i needed a couple of tools to start hacking.
1) arduino - microcontroller to build prototypes: $20.70
2) radio frequency receiver that's listening at the 433MHz band: $7.99
3) led strip - the light: $16.99
4) relay to let electric current flow programmatically: $8.69
5) ac battery pack power supply: $99.99
wait, you're joking about the battery pack right?
please don't clown me.
how's that price comparison looking? $154.36 (openlight) vs. $167.76 (4x the 3 motion sensor lights from walmart) - hey we're under! PLEASE DON'T CLOWN ME
step 3: let’s start listening
first, i wired up the arduino and radio frequency receiver,
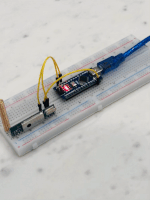
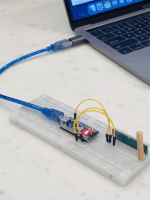
then wrote a little bit of code to output the radio frequency receiver's information to the serial console
int rfPin = 11;
int read_val = 0;
void setup() {
Serial.begin(9600);
pinMode(rfPin, INPUT);
Serial.println("Begin");
}
void loop() {
read_val = digitalRead(rfPin);
Serial.print(read_val);
}
and lastly, i read/tried to interpret the incoming information when separating the two entry sensor pieces.

do you see all of those '1's printed followed by a group of '0's printed? that occurred when the entry sensor's trigger tripped. but what does it mean and how can we use this information to set the door state?
step 4: get frustrated, throw your computer off of the roof, rage quit
step 5: boot, rally, more soco
step 6: whole lotta zeros
the '1's and '0's are the rf receiver's way of transmitting analog input from the real world into the digital world. while the entry sensor may be transmitting encoded information to the base station, instead of trying to decode this data, we can simply look for the encrypted package and treat that as our state flag. so instead of decrypting we intercept and set state variables on our microcontroller.
range bound pickup
after several tests i determined that the number of '0's i would be looking for ranges from 10 to 65, anything over that is not being transmitted by the entry sensor and anything under that is just noise. is it the most elegant solution? certainly not, but remember this is a clown free zone. with code in hand its time to solder a board together and get this hardware/software operational!
int rfPin = 11; // Radio Frequency module set to Pin 11
int relayPin = 3;
int light_state = 0; // Current state of the light, 0 means Off; 1 means On
const int min_off_threshold = 30; // Number of low reads need to be counted to signal entry sensor fired (after min_on_threshold met)
const int max_off_threshold = 65; // Max bumber of low reads or out of bounds
int off_iter = 0;
int prev_val = 0;
int read_val = 0;
int off_reads = 0;
unsigned long fire_time = 0;
void setup() {
Serial.begin(9600);
pinMode(rfPin, INPUT); // sets the digital pin 11 as input
pinMode(relayPin, OUTPUT);
}
void loop() {
read_val = digitalRead(rfPin); // read the input pin
if (read_val == LOW and prev_val == LOW) {
off_iter = off_iter + 1;
} else {
if (off_iter > min_off_threshold and off_iter < max_off_threshold) {
if (millis() - fire_time >= 3000) {
light_state = !light_state;
off_reads = 0;
digitalWrite(relayPin, light_state);
}
off_reads = off_reads + 1;
if (millis() - fire_time < 3000 and off_reads > 3) {
light_state = !light_state;
off_reads = 0;
digitalWrite(relayPin, light_state);
}
fire_time = millis();
}
off_iter = 0;
}
prev_val = read_val;
delay(10);
}
the result
microcontroller, lights, and receiver
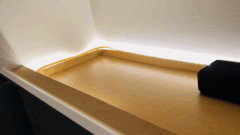
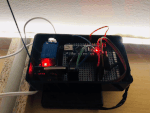
entry sensors
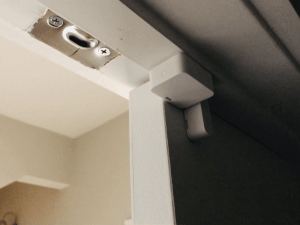
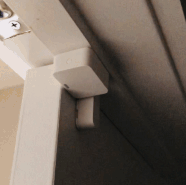
enfin, voila
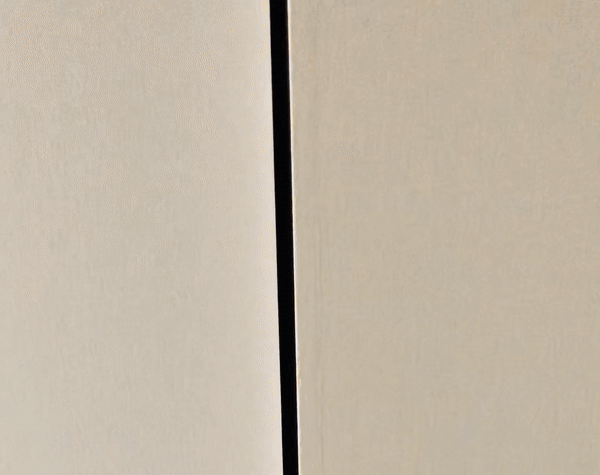
weird flex? but ok.